OneJS also comes with a register()
function that lets you create custom inspectors for MonoBehaviours, ScriptableObjects, and EditorWindows using JS. The current workflow is very experimental.
Sample Usage
Anyhow, here’s a quick example of how to use register()
to create a custom inspector for a MonoBehaviour.
using UnityEngine;
public class Foobar : MonoBehaviour {
public int count;
}
using OneJS.Editor;
using UnityEditor;
using UnityEngine.UIElements;
[CustomEditor(typeof(Foobar))]
public class FoobarEditor : InspectorEditor {
public StyleSheet foobarUSS;
protected override void OnHeaderGUI() {
// Needs this to be empty to prevent the default header from being drawn
}
public override VisualElement CreateInspectorGUI() {
var root = base.CreateInspectorGUI();
// AddInspectorUSS(foobarUSS); // <- can add your static USS here
return root;
}
}
Make sure to drag your EditorScriptEngine
ScriptableObject onto the Engine
field of the FoobarEditor
script (yes you can drag project assets onto a C# script to set some defaults).
declare namespace CS {
class Foobar extends UnityEngine.MonoBehaviour {
public count: number
}
class FoobarEditor extends OneJS.Editor.InspectorEditor { }
}
import { h } from "preact"
import { register, RegisterProps } from "onejs-editor"
import { useCallback, useState } from "preact/hooks"
register(CS.FoobarEditor, ({ target, root }: RegisterProps<CS.FoobarEditor>) => {
const foobar = target.target as CS.Foobar
const [, updateState] = useState({})
const forceUpdate = useCallback(() => updateState({}), [])
function increment() {
foobar.count++
forceUpdate()
}
return <div>
<div class="text-2xl p-3">{`Countx: ${foobar.count}`}</div>
<button class="text-2xl p-3" onClick={increment}>Increment</button>
</div>
})
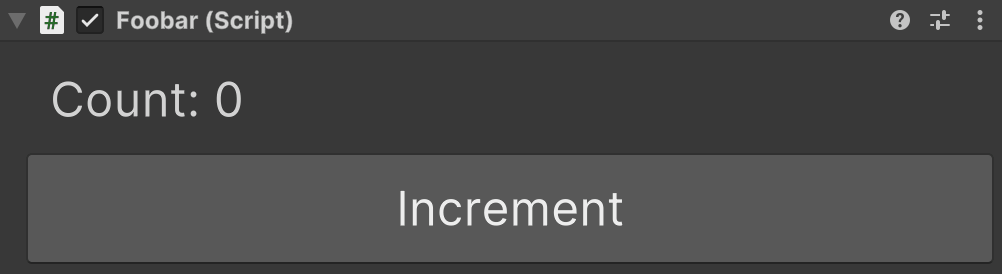
More Thoughts
Keep in mind that the Unity Editor is closely tied to C# and its serialization system, so manually handling things like data syncing and Undo/Redo in JS might not be everyone’s cup of tea.
That said, we can definitely make the workflow smoother by auto-generating the Editor code (like the FoobarEditor.cs
file in the example above), and even make SerializedObject
reactive on the JS side. If that sounds like something you’d be into, hit us up on Discord !