A Basic Example
The script for this example is also available at {ProjectDir}/OneJS/Samples/basic.tsx
. To test it, you can change Live Reload
component's Entry Script
field to Samples/basic.js
(note: this is always a .js file), then go into Playmode.
OneJS has 1 to 1 interop between Preact and UI Toolkit. All controls from UI Toolkit can be accessed from your JSX. The following sample code should be self-explanatory. And as you can see, you can use Preact here pretty normally.
import { h, render } from "preact"
const App = () => {
return (
<div>
<label text="Select something to remove from your suitcase:" />
<box>
<toggle name="boots" label="Boots" value={true} />
<toggle name="helmet" label="Helmet" value={false} />
<toggle name="cloak" label="Cloak of invisibility" />
</box>
<box>
<button name="cancel" text="Cancel" onClick={(e) => log(performance.now())} />
<button name="ok" text="OK" />
<textfield onInput={(e) => log(e.newData)} />
</box>
</div>
)
}
render(<App />, document.body)
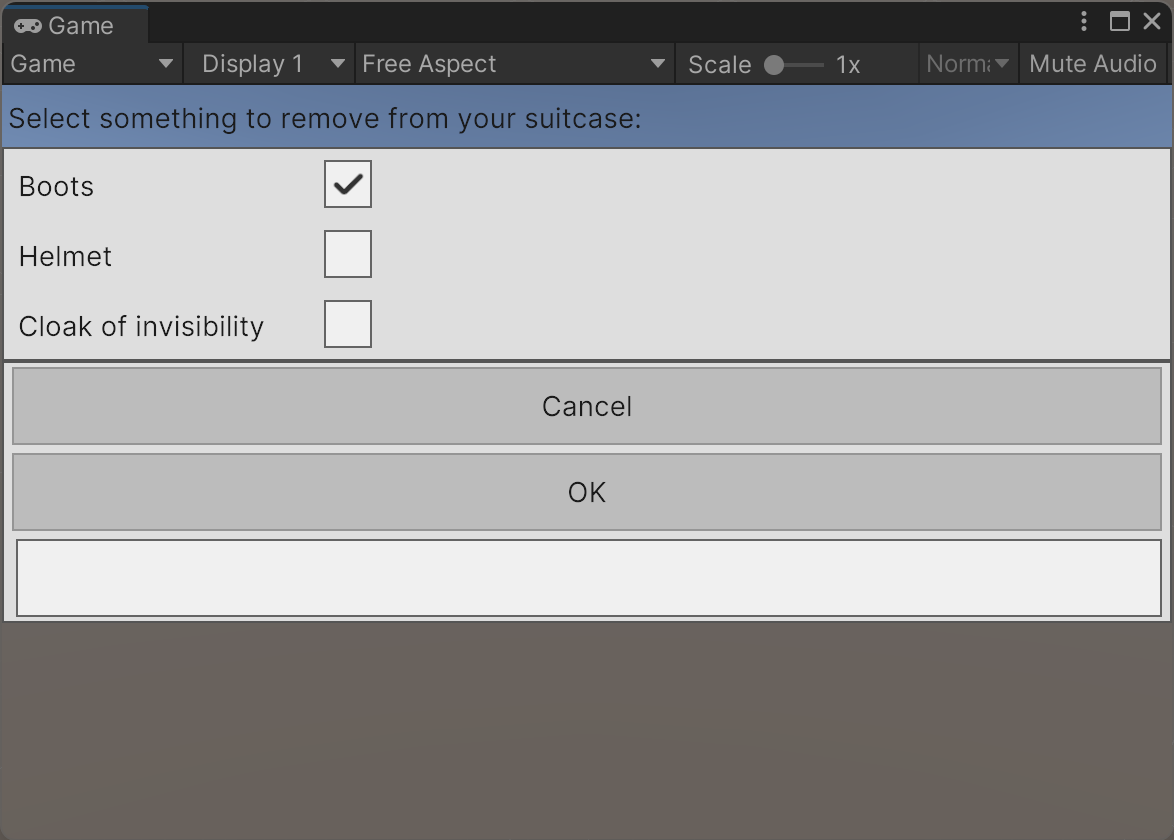
Here's a quick list of UI Toolkit's default controls:
export interface IntrinsicElements {
div: VisualElement
box: Box
text: TextElement
label: Label
image: Image
foldout: Foldout
template: Template
instance: Instance
templatecontainer: TemplateContainer
button: Button
repeatbutton: RepeatButton
toggle: Toggle
scroller: Scroller
slider: Slider
sliderint: SliderInt
minmaxslider: MinMaxSlider
enumfield: EnumField
progressbar: ProgressBar
textfield: TextField
integerfield: IntegerField
floatfield: FloatField
vector2field: Vector2Field
vector2intfield: Vector2IntField
vector3field: Vector3Field
vector3intfield: Vector3IntField
vector4field: Vector4Field
rectfield: RectField
rectintfield: RectIntField
boundsfield: BoundsField
boundsintfield: BoundsIntField
listview: ListView
scrollview: ScrollView
treeview: TreeView
popupwindow: PopupWindow
gradientrect: GradientRect
flipbook: Flipbook
simplelistview: SimpleListView
}